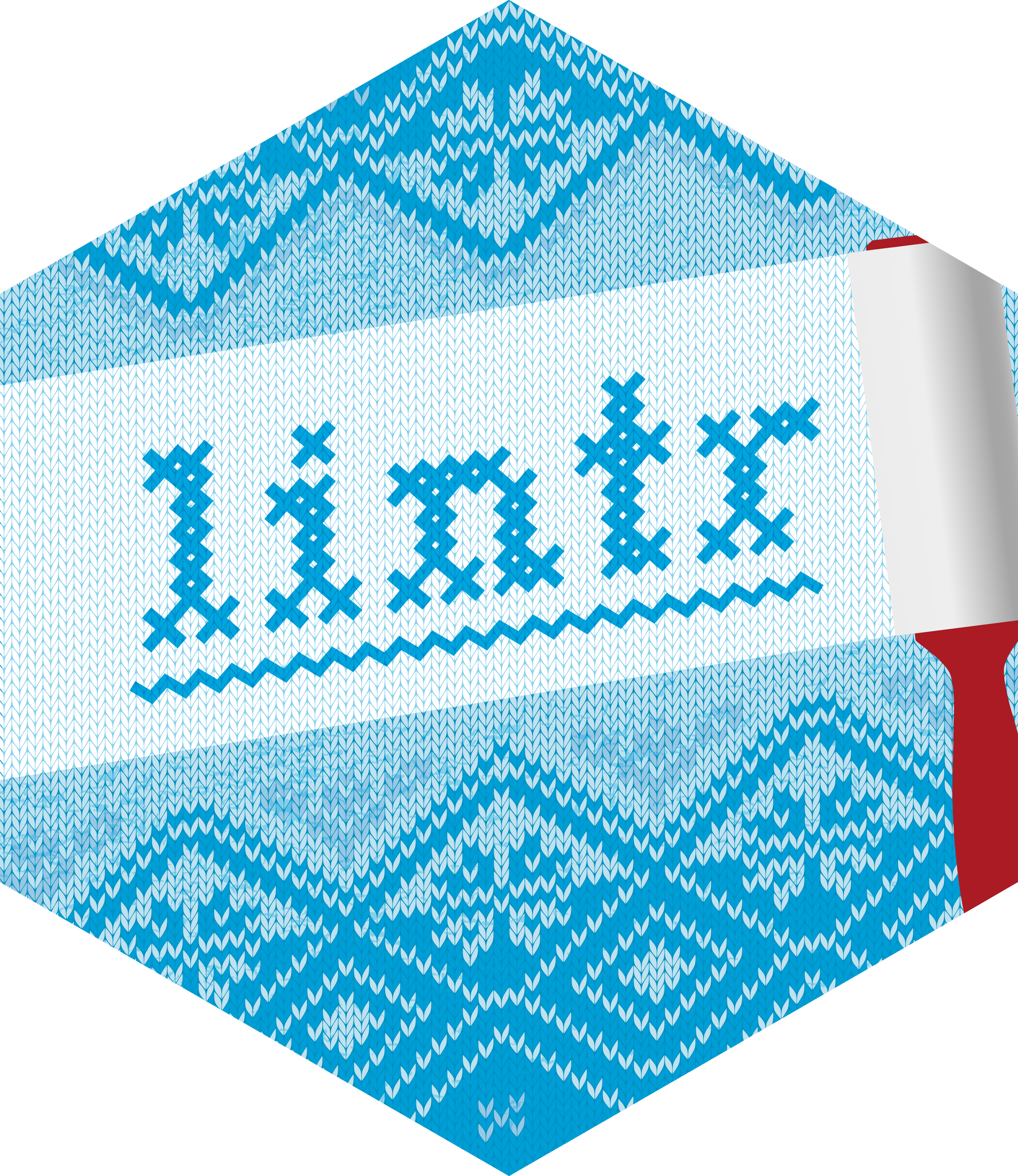
Lint common mistakes/style issues cropping up from return statements
Source:R/function_return_linter.R
function_return_linter.Rd
return(x <- ...)
is either distracting (because x
is ignored), or
confusing (because assigning to x
has some side effect that is muddled
by the dual-purpose expression).
See also
linters for a complete list of linters available in lintr.
Examples
# will produce lints
lint(
text = "foo <- function(x) return(y <- x + 1)",
linters = function_return_linter()
)
#> <text>:1:27: warning: [function_return_linter] Move the assignment outside of the return() clause, or skip assignment altogether.
#> foo <- function(x) return(y <- x + 1)
#> ^~~~~~~~~~
lint(
text = "foo <- function(x) return(x <<- x + 1)",
linters = function_return_linter()
)
#> <text>:1:27: warning: [function_return_linter] Move the assignment outside of the return() clause, or skip assignment altogether.
#> foo <- function(x) return(x <<- x + 1)
#> ^~~~~~~~~~~
writeLines("e <- new.env() \nfoo <- function(x) return(e$val <- x + 1)")
#> e <- new.env()
#> foo <- function(x) return(e$val <- x + 1)
lint(
text = "e <- new.env() \nfoo <- function(x) return(e$val <- x + 1)",
linters = function_return_linter()
)
#> <text>:2:27: warning: [function_return_linter] Move the assignment outside of the return() clause, or skip assignment altogether.
#> foo <- function(x) return(e$val <- x + 1)
#> ^~~~~~~~~~~~~~
# okay
lint(
text = "foo <- function(x) return(x + 1)",
linters = function_return_linter()
)
#> ℹ No lints found.
code_lines <- "
foo <- function(x) {
x <<- x + 1
return(x)
}
"
lint(
text = code_lines,
linters = function_return_linter()
)
#> ℹ No lints found.
code_lines <- "
e <- new.env()
foo <- function(x) {
e$val <- x + 1
return(e$val)
}
"
writeLines(code_lines)
#>
#> e <- new.env()
#> foo <- function(x) {
#> e$val <- x + 1
#> return(e$val)
#> }
#>
lint(
text = code_lines,
linters = function_return_linter()
)
#> ℹ No lints found.